swagger
本文作者:小雷FansUnion-一个有创业和投资经验的资深程序员-全球最大中文IT社区CSDN知名博主-排名第119 实际项目中非常需要写文档,提高Java服务端和Web前端以及移动端的对接效率。 听说Swagger这个工具,还不错,就网上找了些资料,自己实践了下。一:Swagger介绍Swagger是当前最好用的Restful API文档生成的...
一:Swagger介绍
Swagger是当前最好用的Restful API文档生成的开源项目,通过swagger-spring项目
实现了与SpingMVC框架的无缝集成功能,方便生成spring restful风格的接口文档,
同时swagger-ui还可以测试spring restful风格的接口功能。
官方网站为:http://swagger.io/
-
<dependency>
-
<groupId>com.mangofactory
</groupId>
-
<artifactId>swagger-springmvc
</artifactId>
-
<version>1.0.2
</version>
-
</dependency>
-
-
<dependency>
-
<groupId>org.springframework
</groupId>
-
<artifactId>spring-webmvc
</artifactId>
-
<version>4.1.6.RELEASE
</version>
-
</dependency>
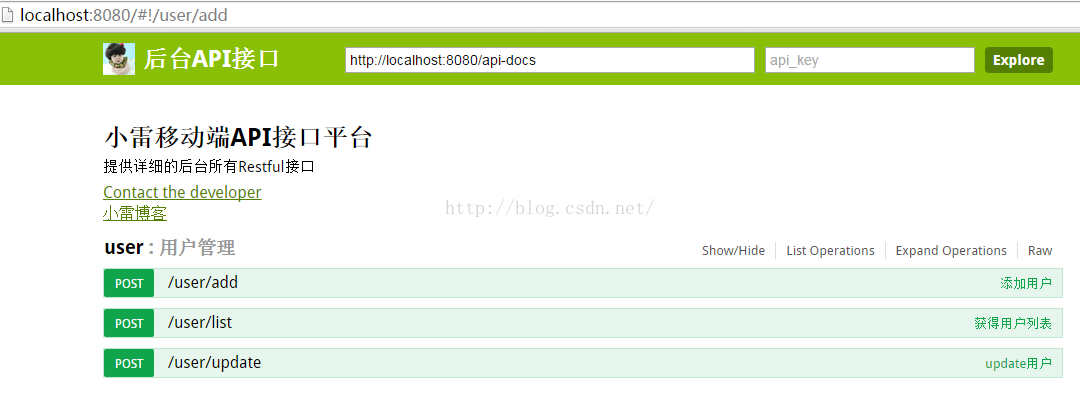
@Api:修饰整个类,描述Controller的作用
@ApiOperation:描述一个类的一个方法,或者说一个接口
@ApiParam:单个参数描述
@ApiModel:用对象来接收参数
@ApiProperty:用对象接收参数时,描述对象的一个字段
其它若干
@ApiResponse:HTTP响应其中1个描述
@ApiResponses:HTTP响应整体描述
@ApiClass
@ApiError
@ApiErrors
@ApiParamImplicit
@ApiParamsImplicit
-
@ApiOperation(value =
"获得用户列表", notes =
"列表信息", httpMethod =
"POST", produces = MediaType.APPLICATION_JSON_VALUE)
-
@ResponseBody
-
@RequestMapping(value =
"list", method = RequestMethod.POST)
-
public Result<User> list(
-
@ApiParam(value = "分类ID", required = true) @RequestParam Long categoryId,
-
@ApiParam(value = "token", required = true) @RequestParam String token) {
-
Result<User> result =
new Result<User>();
-
User user =
new User();
-
result.setData(user);
-
return result;
-
}
-
@ApiOperation(value =
"update用户", notes =
")", httpMethod =
"POST", produces = MediaType.APPLICATION_JSON_VALUE)
-
@ResponseBody
-
@RequestMapping(value =
"update", method = RequestMethod.GET
/*,produces = MediaType.APPLICATION_FORM_URLENCODED_VALUE*/)
-
public Result<String> update(User user) {
-
String u = findUser(user);
-
System.out.println(u);
-
return
null;
-
}
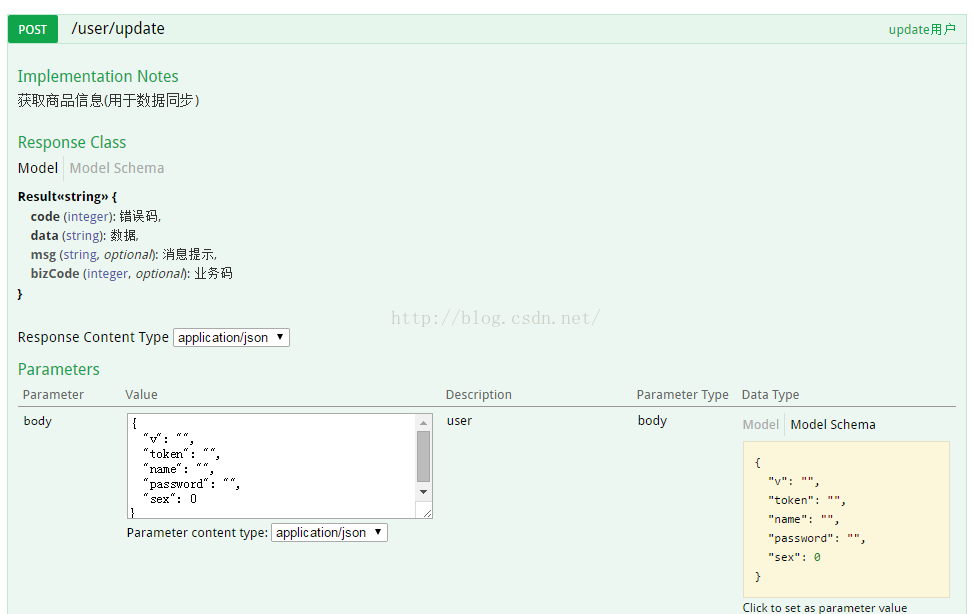
-
public Result<String> add(@RequestBody User user) {
-
String u = findUser(user);
-
System.out.println(u);
-
return
null;
-
}
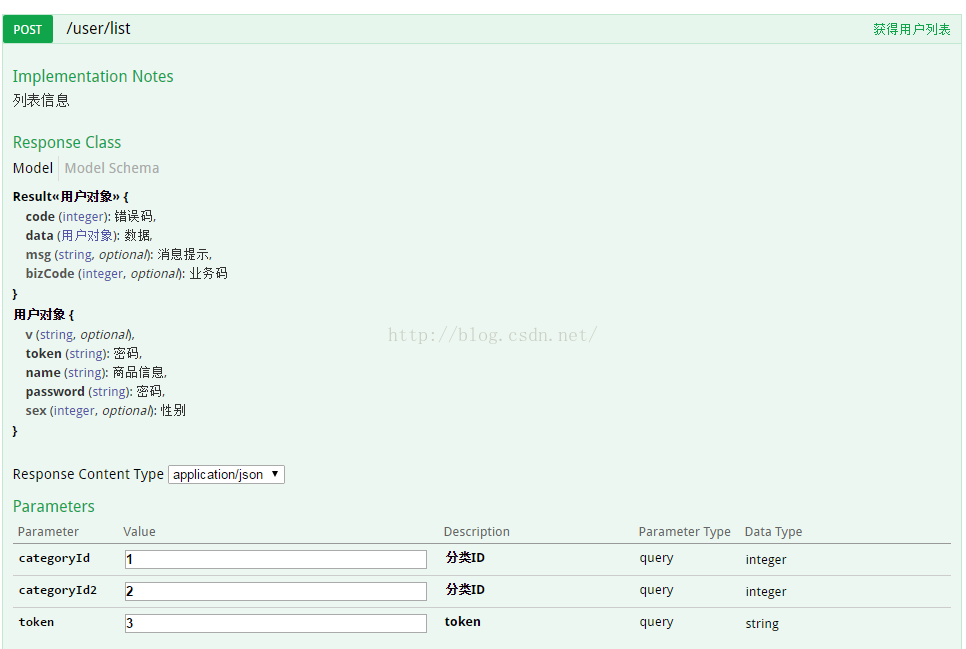
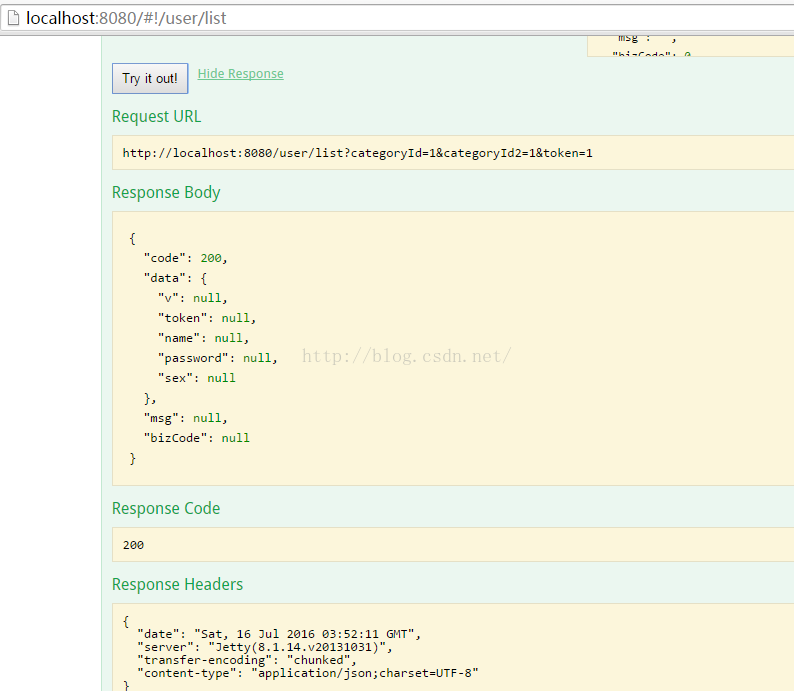
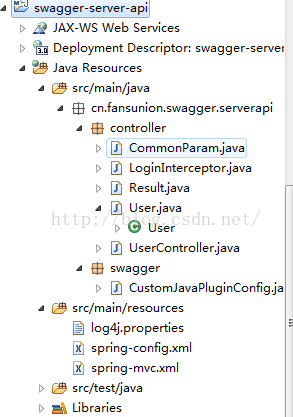
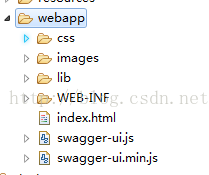
-
package cn.fansunion.swagger.serverapi.controller;
-
-
import org.springframework.http.MediaType;
-
import org.springframework.stereotype.Controller;
-
import org.springframework.web.bind.annotation.RequestBody;
-
import org.springframework.web.bind.annotation.RequestMapping;
-
import org.springframework.web.bind.annotation.RequestMethod;
-
import org.springframework.web.bind.annotation.RequestParam;
-
import org.springframework.web.bind.annotation.ResponseBody;
-
-
import com.wordnik.swagger.annotations.Api;
-
import com.wordnik.swagger.annotations.ApiOperation;
-
import com.wordnik.swagger.annotations.ApiParam;
-
-
/**
-
* 小雷FansUnion-一个有创业和投资经验的资深程序员-全球最大中文IT社区CSDN知名博主-排名第119
-
* 博客:http://blog.csdn.net/fansunion
-
*
-
*/
-
@Api(value =
"user", description =
"用户管理", produces = MediaType.APPLICATION_JSON_VALUE)
-
@Controller
-
@RequestMapping(
"user")
-
public
class UserController {
-
-
// 列出某个类目的所有规格
-
@ApiOperation(value =
"获得用户列表", notes =
"列表信息", httpMethod =
"POST", produces = MediaType.APPLICATION_JSON_VALUE)
-
@ResponseBody
-
@RequestMapping(value =
"list", method = RequestMethod.POST)
-
public Result<User> list(
-
@ApiParam(value = "分类ID", required = true) @RequestParam Long categoryId,
-
@ApiParam(value = "分类ID", required = true) @RequestParam Long categoryId2,
-
@ApiParam(value = "token", required = true) @RequestParam String token) {
-
Result<User> result =
new Result<User>();
-
User user =
new User();
-
result.setData(user);
-
return result;
-
}
-
-
@ApiOperation(value =
"添加用户", notes =
"获取商品信息(用于数据同步)", httpMethod =
"POST", produces = MediaType.APPLICATION_JSON_VALUE)
-
@ResponseBody
-
@RequestMapping(value =
"add", method = RequestMethod.POST)
-
// @RequestBody只能有1个
-
// 使用了@RequestBody,不能在拦截器中,获得流中的数据,再json转换,拦截器中,也不清楚数据的类型,无法转换成java对象
-
// 只能手动调用方法
-
public Result<String> add(@RequestBody User user) {
-
String u = findUser(user);
-
System.out.println(u);
-
return
null;
-
}
-
-
@ApiOperation(value =
"update用户", notes =
"获取商品信息(用于数据同步)", httpMethod =
"POST", produces = MediaType.APPLICATION_JSON_VALUE)
-
@ResponseBody
-
@RequestMapping(value =
"update", method = RequestMethod.GET)
-
public Result<String> update(User user) {
-
String u = findUser(user);
-
System.out.println(u);
-
return
null;
-
}
-
-
private String findUser(User user) {
-
String token = user.getToken();
-
return token;
-
}
-
}
-
package cn.fansunion.swagger.serverapi.controller;
-
-
import com.wordnik.swagger.annotations.ApiModel;
-
import com.wordnik.swagger.annotations.ApiModelProperty;
-
-
/**
-
* 小雷FansUnion-一个有创业和投资经验的资深程序员-全球最大中文IT社区CSDN知名博主-排名第119
-
* 博客:http://blog.csdn.net/fansunion
-
*
-
*/
-
@ApiModel(value =
"用户对象", description =
"user2")
-
public
class User extends CommonParam {
-
-
@ApiModelProperty(value =
"商品信息", required =
true)
-
private String name;
-
@ApiModelProperty(value =
"密码", required =
true)
-
private String password;
-
-
@ApiModelProperty(value =
"性别")
-
private Integer sex;
-
@ApiModelProperty(value =
"密码", required =
true)
-
private String token;
-
-
public String getToken() {
-
return token;
-
}
-
-
public void setToken(String token) {
-
this.token = token;
-
}
-
-
public String getName() {
-
return name;
-
}
-
-
public void setName(String name) {
-
this.name = name;
-
}
-
-
public String getPassword() {
-
return password;
-
}
-
-
public void setPassword(String password) {
-
this.password = password;
-
}
-
-
public Integer getSex() {
-
return sex;
-
}
-
-
public void setSex(Integer sex) {
-
this.sex = sex;
-
}
-
-
}
-
package cn.fansunion.swagger.serverapi.swagger;
-
-
import org.springframework.beans.factory.annotation.Autowired;
-
import org.springframework.context.annotation.Bean;
-
import org.springframework.context.annotation.Configuration;
-
import org.springframework.web.servlet.config.annotation.DefaultServletHandlerConfigurer;
-
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
-
import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter;
-
-
import com.mangofactory.swagger.configuration.SpringSwaggerConfig;
-
import com.mangofactory.swagger.models.dto.ApiInfo;
-
import com.mangofactory.swagger.paths.SwaggerPathProvider;
-
import com.mangofactory.swagger.plugin.EnableSwagger;
-
import com.mangofactory.swagger.plugin.SwaggerSpringMvcPlugin;
-
-
@Configuration
-
@EnableWebMvc
-
@EnableSwagger
-
public
class CustomJavaPluginConfig extends WebMvcConfigurerAdapter {
-
-
private SpringSwaggerConfig springSwaggerConfig;
-
-
@Autowired
-
public void setSpringSwaggerConfig(SpringSwaggerConfig springSwaggerConfig) {
-
this.springSwaggerConfig = springSwaggerConfig;
-
}
-
-
/**
-
* 链式编程 来定制API样式 后续会加上分组信息
-
*
-
* @return
-
*/
-
@Bean
-
public SwaggerSpringMvcPlugin customImplementation() {
-
return
new SwaggerSpringMvcPlugin(
this.springSwaggerConfig)
-
.apiInfo(apiInfo()).includePatterns(
".*")
-
.useDefaultResponseMessages(
false)
-
// .pathProvider(new GtPaths())
-
.apiVersion(
"0.1").swaggerGroup(
"user");
-
-
}
-
-
private ApiInfo apiInfo() {
-
ApiInfo apiInfo =
new ApiInfo(
"小雷移动端API接口平台",
-
"提供详细的后台所有Restful接口",
"http://blog.csdn.net/FansUnion",
-
"FansUnion@qq.com",
"小雷博客",
"http://blog.csdn.net/FansUnion");
-
return apiInfo;
-
}
-
-
@Override
-
public void configureDefaultServletHandling(
-
DefaultServletHandlerConfigurer configurer) {
-
configurer.enable();
-
}
-
-
class GtPaths extends SwaggerPathProvider {
-
-
@Override
-
protected String applicationPath() {
-
return
"/restapi";
-
}
-
-
@Override
-
protected String getDocumentationPath() {
-
return
"/restapi";
-
}
-
}
-
-
}
一:Swagger介绍
Swagger是当前最好用的Restful API文档生成的开源项目,通过swagger-spring项目
实现了与SpingMVC框架的无缝集成功能,方便生成spring restful风格的接口文档,
同时swagger-ui还可以测试spring restful风格的接口功能。
官方网站为:http://swagger.io/
-
<dependency>
-
<groupId>com.mangofactory
</groupId>
-
<artifactId>swagger-springmvc
</artifactId>
-
<version>1.0.2
</version>
-
</dependency>
-
-
<dependency>
-
<groupId>org.springframework
</groupId>
-
<artifactId>spring-webmvc
</artifactId>
-
<version>4.1.6.RELEASE
</version>
-
</dependency>
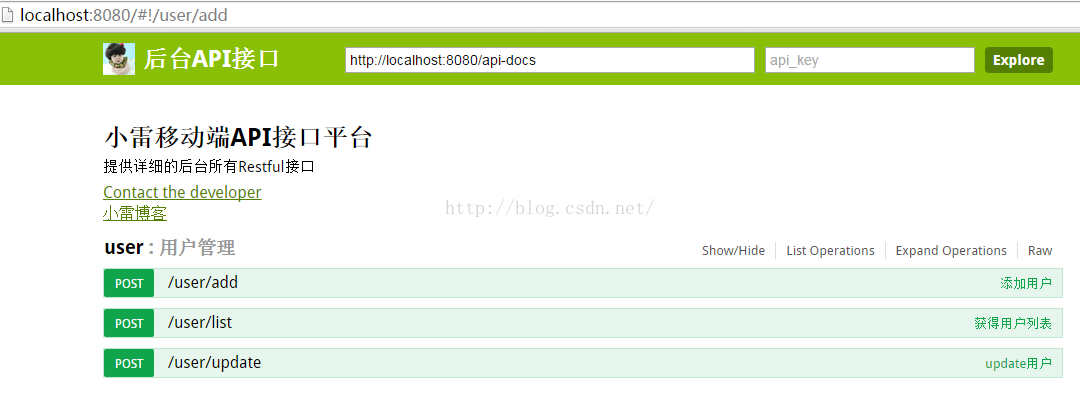
@Api:修饰整个类,描述Controller的作用
@ApiOperation:描述一个类的一个方法,或者说一个接口
@ApiParam:单个参数描述
@ApiModel:用对象来接收参数
@ApiProperty:用对象接收参数时,描述对象的一个字段
其它若干
@ApiResponse:HTTP响应其中1个描述
@ApiResponses:HTTP响应整体描述
@ApiClass
@ApiError
@ApiErrors
@ApiParamImplicit
@ApiParamsImplicit
-
@ApiOperation(value =
"获得用户列表", notes =
"列表信息", httpMethod =
"POST", produces = MediaType.APPLICATION_JSON_VALUE)
-
@ResponseBody
-
@RequestMapping(value =
"list", method = RequestMethod.POST)
-
public Result<User> list(
-
@ApiParam(value = "分类ID", required = true) @RequestParam Long categoryId,
-
@ApiParam(value = "token", required = true) @RequestParam String token) {
-
Result<User> result =
new Result<User>();
-
User user =
new User();
-
result.setData(user);
-
return result;
-
}
-
@ApiOperation(value =
"update用户", notes =
")", httpMethod =
"POST", produces = MediaType.APPLICATION_JSON_VALUE)
-
@ResponseBody
-
@RequestMapping(value =
"update", method = RequestMethod.GET
/*,produces = MediaType.APPLICATION_FORM_URLENCODED_VALUE*/)
-
public Result<String> update(User user) {
-
String u = findUser(user);
-
System.out.println(u);
-
return
null;
-
}
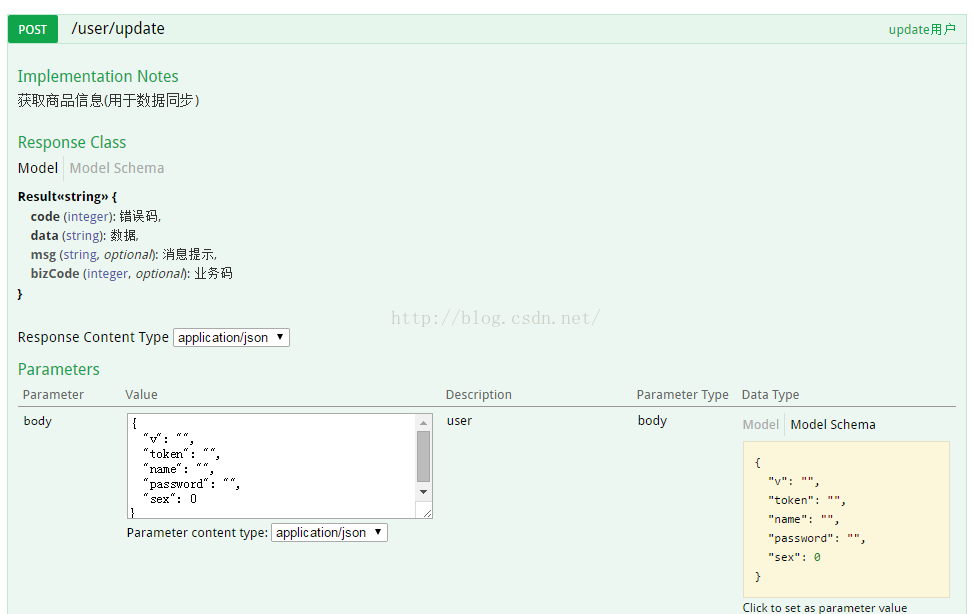
-
public Result<String> add(@RequestBody User user) {
-
String u = findUser(user);
-
System.out.println(u);
-
return
null;
-
}
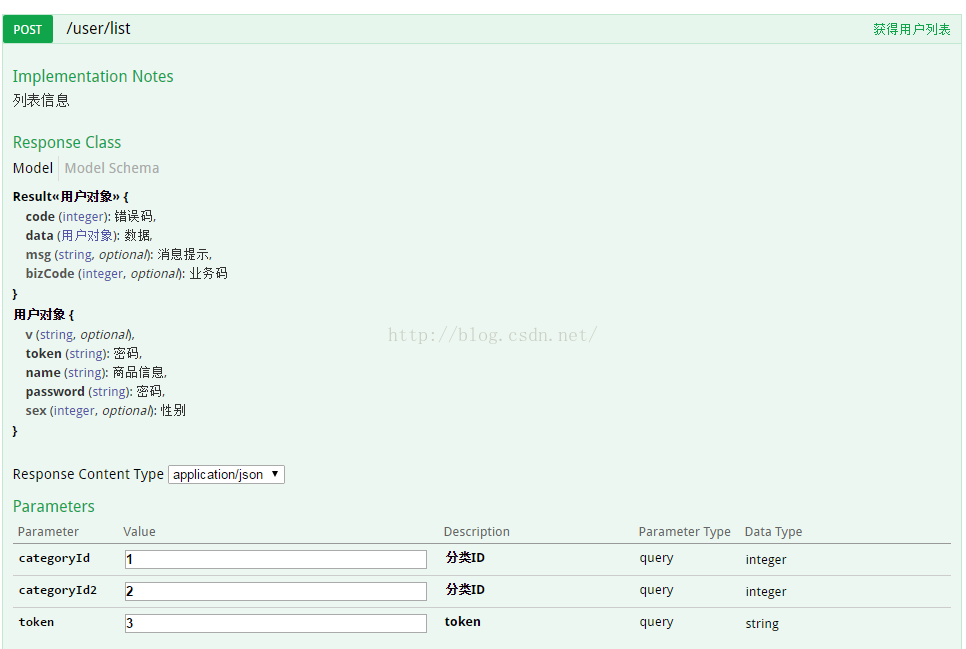
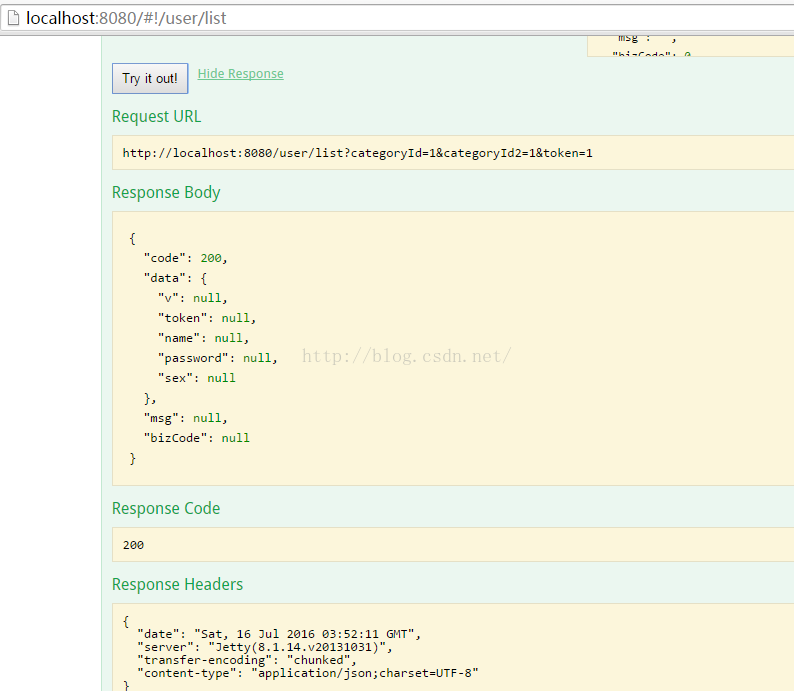
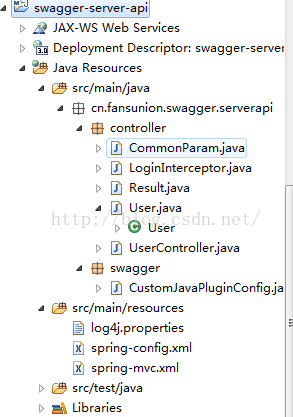
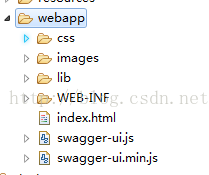
-
package cn.fansunion.swagger.serverapi.controller;
-
-
import org.springframework.http.MediaType;
-
import org.springframework.stereotype.Controller;
-
import org.springframework.web.bind.annotation.RequestBody;
-
import org.springframework.web.bind.annotation.RequestMapping;
-
import org.springframework.web.bind.annotation.RequestMethod;
-
import org.springframework.web.bind.annotation.RequestParam;
-
import org.springframework.web.bind.annotation.ResponseBody;
-
-
import com.wordnik.swagger.annotations.Api;
-
import com.wordnik.swagger.annotations.ApiOperation;
-
import com.wordnik.swagger.annotations.ApiParam;
-
-
/**
-
* 小雷FansUnion-一个有创业和投资经验的资深程序员-全球最大中文IT社区CSDN知名博主-排名第119
-
* 博客:http://blog.csdn.net/fansunion
-
*
-
*/
-
@Api(value =
"user", description =
"用户管理", produces = MediaType.APPLICATION_JSON_VALUE)
-
@Controller
-
@RequestMapping(
"user")
-
public
class UserController {
-
-
// 列出某个类目的所有规格
-
@ApiOperation(value =
"获得用户列表", notes =
"列表信息", httpMethod =
"POST", produces = MediaType.APPLICATION_JSON_VALUE)
-
@ResponseBody
-
@RequestMapping(value =
"list", method = RequestMethod.POST)
-
public Result<User> list(
-
@ApiParam(value = "分类ID", required = true) @RequestParam Long categoryId,
-
@ApiParam(value = "分类ID", required = true) @RequestParam Long categoryId2,
-
@ApiParam(value = "token", required = true) @RequestParam String token) {
-
Result<User> result =
new Result<User>();
-
User user =
new User();
-
result.setData(user);
-
return result;
-
}
-
-
@ApiOperation(value =
"添加用户", notes =
"获取商品信息(用于数据同步)", httpMethod =
"POST", produces = MediaType.APPLICATION_JSON_VALUE)
-
@ResponseBody
-
@RequestMapping(value =
"add", method = RequestMethod.POST)
-
// @RequestBody只能有1个
-
// 使用了@RequestBody,不能在拦截器中,获得流中的数据,再json转换,拦截器中,也不清楚数据的类型,无法转换成java对象
-
// 只能手动调用方法
-
public Result<String> add(@RequestBody User user) {
-
String u = findUser(user);
-
System.out.println(u);
-
return
null;
-
}
-
-
@ApiOperation(value =
"update用户", notes =
"获取商品信息(用于数据同步)", httpMethod =
"POST", produces = MediaType.APPLICATION_JSON_VALUE)
-
@ResponseBody
-
@RequestMapping(value =
"update", method = RequestMethod.GET)
-
public Result<String> update(User user) {
-
String u = findUser(user);
-
System.out.println(u);
-
return
null;
-
}
-
-
private String findUser(User user) {
-
String token = user.getToken();
-
return token;
-
}
-
}
-
package cn.fansunion.swagger.serverapi.controller;
-
-
import com.wordnik.swagger.annotations.ApiModel;
-
import com.wordnik.swagger.annotations.ApiModelProperty;
-
-
/**
-
* 小雷FansUnion-一个有创业和投资经验的资深程序员-全球最大中文IT社区CSDN知名博主-排名第119
-
* 博客:http://blog.csdn.net/fansunion
-
*
-
*/
-
@ApiModel(value =
"用户对象", description =
"user2")
-
public
class User extends CommonParam {
-
-
@ApiModelProperty(value =
"商品信息", required =
true)
-
private String name;
-
@ApiModelProperty(value =
"密码", required =
true)
-
private String password;
-
-
@ApiModelProperty(value =
"性别")
-
private Integer sex;
-
@ApiModelProperty(value =
"密码", required =
true)
-
private String token;
-
-
public String getToken() {
-
return token;
-
}
-
-
public void setToken(String token) {
-
this.token = token;
-
}
-
-
public String getName() {
-
return name;
-
}
-
-
public void setName(String name) {
-
this.name = name;
-
}
-
-
public String getPassword() {
-
return password;
-
}
-
-
public void setPassword(String password) {
-
this.password = password;
-
}
-
-
public Integer getSex() {
-
return sex;
-
}
-
-
public void setSex(Integer sex) {
-
this.sex = sex;
-
}
-
-
}
-
package cn.fansunion.swagger.serverapi.swagger;
-
-
import org.springframework.beans.factory.annotation.Autowired;
-
import org.springframework.context.annotation.Bean;
-
import org.springframework.context.annotation.Configuration;
-
import org.springframework.web.servlet.config.annotation.DefaultServletHandlerConfigurer;
-
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
-
import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter;
-
-
import com.mangofactory.swagger.configuration.SpringSwaggerConfig;
-
import com.mangofactory.swagger.models.dto.ApiInfo;
-
import com.mangofactory.swagger.paths.SwaggerPathProvider;
-
import com.mangofactory.swagger.plugin.EnableSwagger;
-
import com.mangofactory.swagger.plugin.SwaggerSpringMvcPlugin;
-
-
@Configuration
-
@EnableWebMvc
-
@EnableSwagger
-
public
class CustomJavaPluginConfig extends WebMvcConfigurerAdapter {
-
-
private SpringSwaggerConfig springSwaggerConfig;
-
-
@Autowired
-
public void setSpringSwaggerConfig(SpringSwaggerConfig springSwaggerConfig) {
-
this.springSwaggerConfig = springSwaggerConfig;
-
}
-
-
/**
-
* 链式编程 来定制API样式 后续会加上分组信息
-
*
-
* @return
-
*/
-
@Bean
-
public SwaggerSpringMvcPlugin customImplementation() {
-
return
new SwaggerSpringMvcPlugin(
this.springSwaggerConfig)
-
.apiInfo(apiInfo()).includePatterns(
".*")
-
.useDefaultResponseMessages(
false)
-
// .pathProvider(new GtPaths())
-
.apiVersion(
"0.1").swaggerGroup(
"user");
-
-
}
-
-
private ApiInfo apiInfo() {
-
ApiInfo apiInfo =
new ApiInfo(
"小雷移动端API接口平台",
-
"提供详细的后台所有Restful接口",
"http://blog.csdn.net/FansUnion",
-
"FansUnion@qq.com",
"小雷博客",
"http://blog.csdn.net/FansUnion");
-
return apiInfo;
-
}
-
-
@Override
-
public void configureDefaultServletHandling(
-
DefaultServletHandlerConfigurer configurer) {
-
configurer.enable();
-
}
-
-
class GtPaths extends SwaggerPathProvider {
-
-
@Override
-
protected String applicationPath() {
-
return
"/restapi";
-
}
-
-
@Override
-
protected String getDocumentationPath() {
-
return
"/restapi";
-
}
-
}
-
-
}
更多推荐
所有评论(0)