微信小程序配置接口调用API
本文介绍小程序调用接口的封装,并通过koa2写个测试接口调用。
·
本文介绍小程序调用
API
接口的封装,并通过koa2
写个测试接口调用。
基本目录
├── api
│ └── api.js // 接口配置
├── pages
│ ├── index // 测试页面
│ │ ├── index.js
│ │ ├── index.json
│ │ ├── index.wxml
│ │ └── index.wxss
├── utils
│ └── util.js
...
./api/api
// 定义基本URL
const BASE_URL = 'http://0.0.0.0:3000';
// 封装请求
const request = (url, method, data) => {
return new Promise((resolve, reject) => {
wx.request({
url: BASE_URL + '/api/v2' + url,
method: method,
data: data || {},
header: {
Accept: "application/json",
'Content-Type': 'application/x-www-form-urlencoded'
},
success(request) {
resolve(request.data)
},
fail(error) {
reject(error)
},
complete(res) {
console.log('loading completed');
}
})
})
}
// 扩展 promise 的 finally 方法
Promise.prototype.finally = function (callback) {
let P = this.constructor;
return this.then(
value => {
P.resolve(callback()).then(() => value);
},
reason => {
P.resolve(callback()).then( () => { throw reason });
}
);
}
// 导出接口
module.exports = {
// 测试接口
test(params){
return request('/test','post', params);
}
}
- 首先定义基础接口路径
BASE_URL
- 封装
request
返回一个用Promise
包裹的wx.request
方法 - 后面扩展了
Promise
实例的finally
方法。原因是手机上会报没有finally
这个方法的错。 - 最后导出接口,此示例导出一个
post
方式请求的test
方法。
测试接口 ./pages/index/index.js 只列出相关代码
// 导入接口
import { test } from '../../api/api';
Page({
data: {
...
res_data: null, // 定义数据
...
},
...
onLoad() {
test({
title: "post-test"
}).then(res=>{
this.setData({
res_data: JSON.stringify(res)
});
})
},
...
})
展示接口数据 ./pages/index/index.wxml
<view class="container">
{{api_test}}
</view>
调用接口后获取到的 json
结构
{
"code":200,
"data":{
"title":"post-test",
"message":"wechat applet api test post"
},
"msg":"success"
}
- 这个
json
结构是由koa2
简单写个示例提供。
koa2
相关代码
const Router = require('koa-router');
const router = new Router();
router.post('/test', async (ctx,next) => {
const { title } = ctx.request.body;
ctx.body = {
code: 200,
data:{
title: title || "default",
message: "wechat applet api test post"
},
msg: "success"
};
});
router.use('/api/v2', router.routes());
module.exports = router;
- 为了简化,直接把返回结果写在路由里。
更多推荐
🚀API 研发管理和自动化测试高效工具
活动日历
查看更多
活动时间 2023-08-23 00:00:00
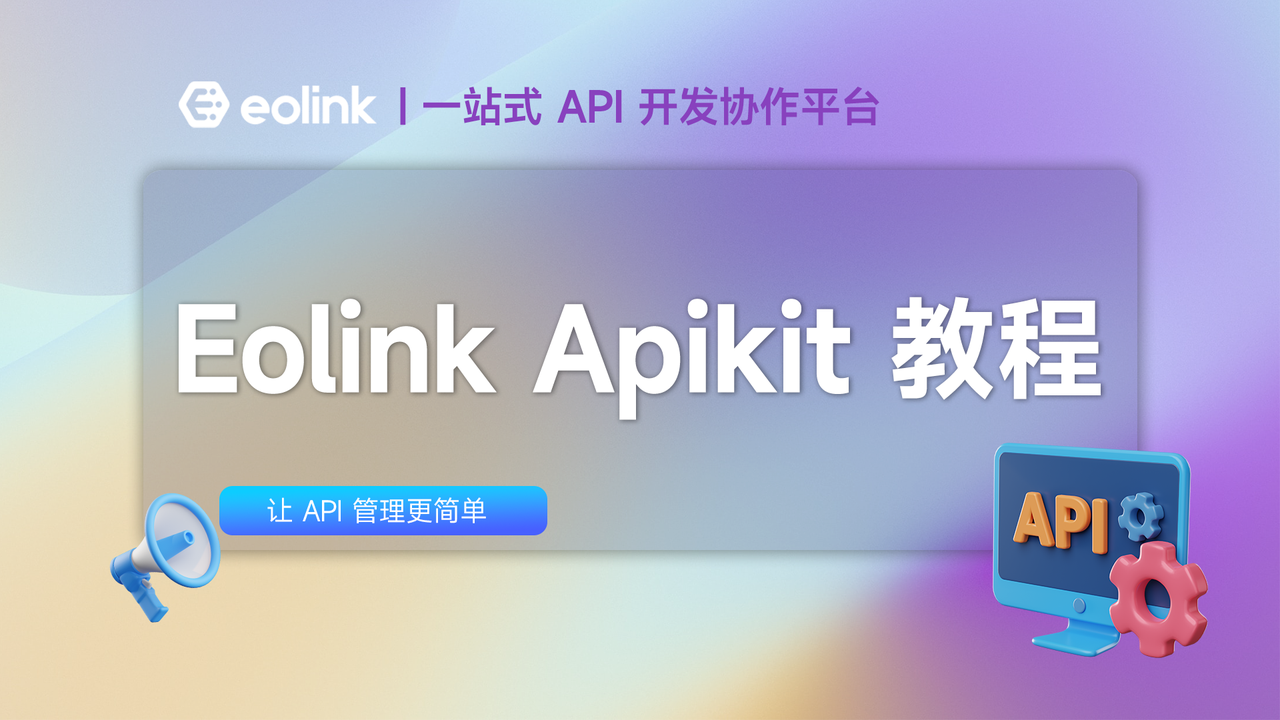
教程|Eolink Apikit 快速入门
活动时间 2023-07-04 00:00:00
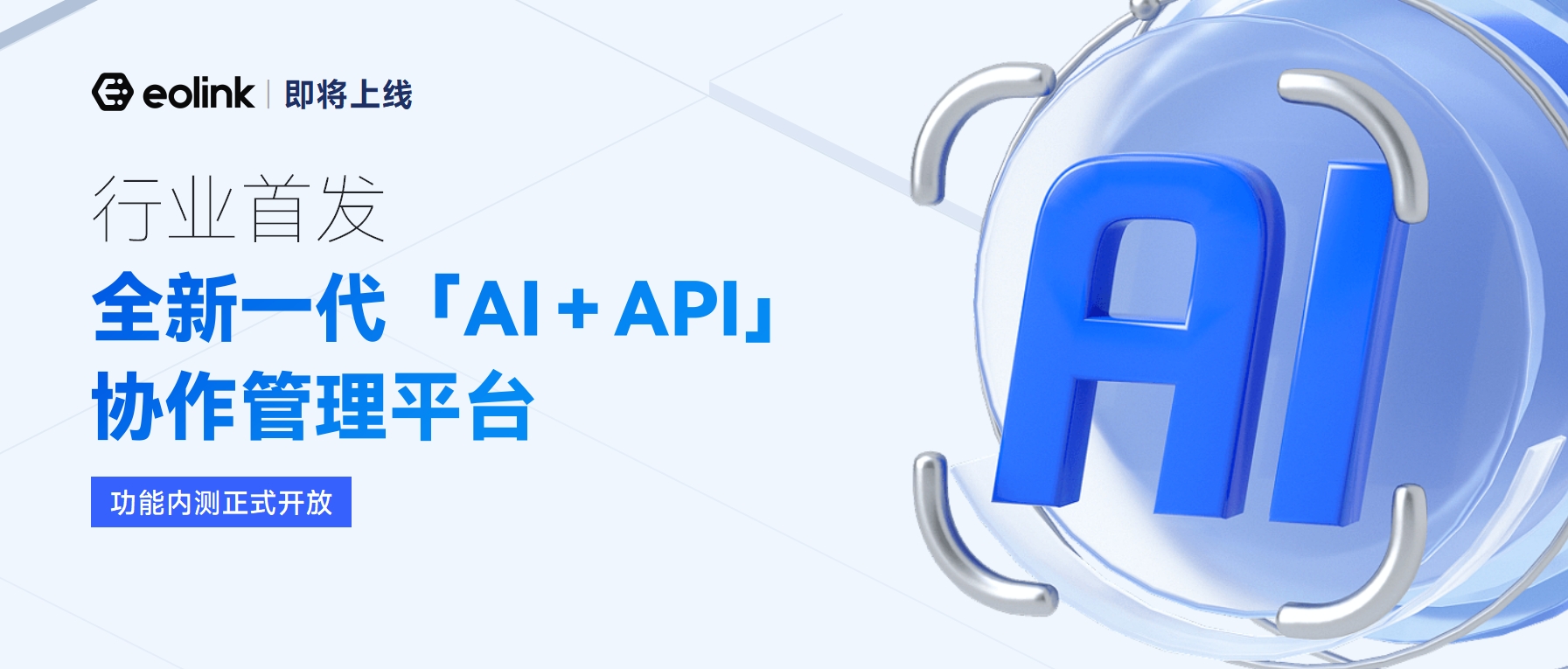
【活动】Eolink「AI+API」新功能正式开放内测!
直播时间 2023-03-23 18:44:14
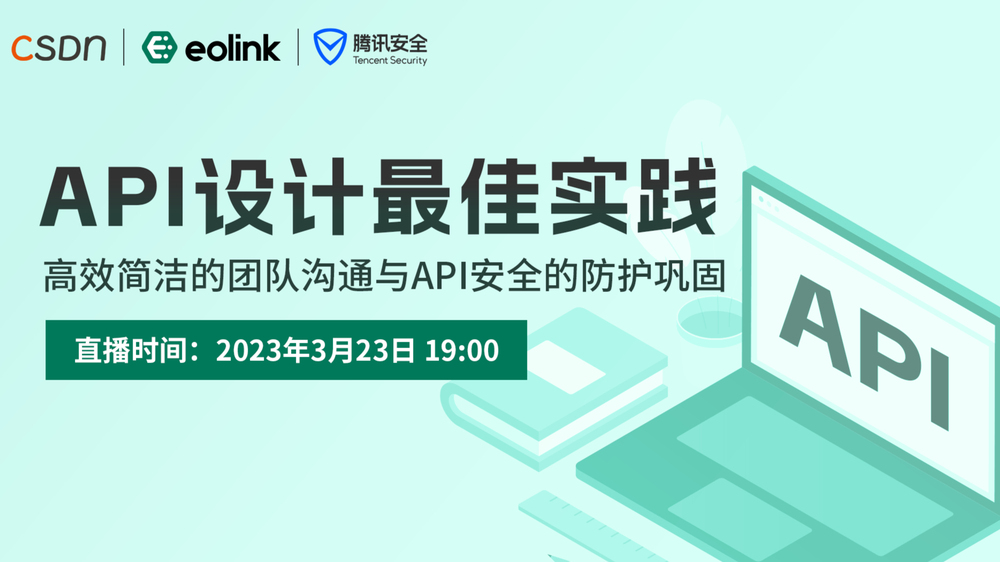
API设计最佳实践|高效简洁的团队沟通与API安全的防护巩固
直播时间 2022-12-15 18:30:18
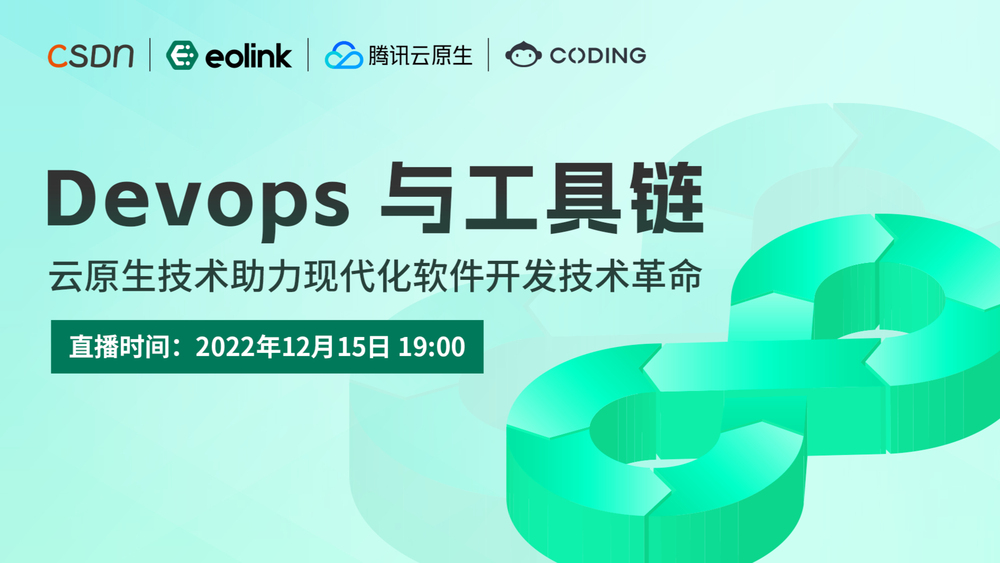
Devops与工具链|云原生技术助力现代化软件开发技术革命
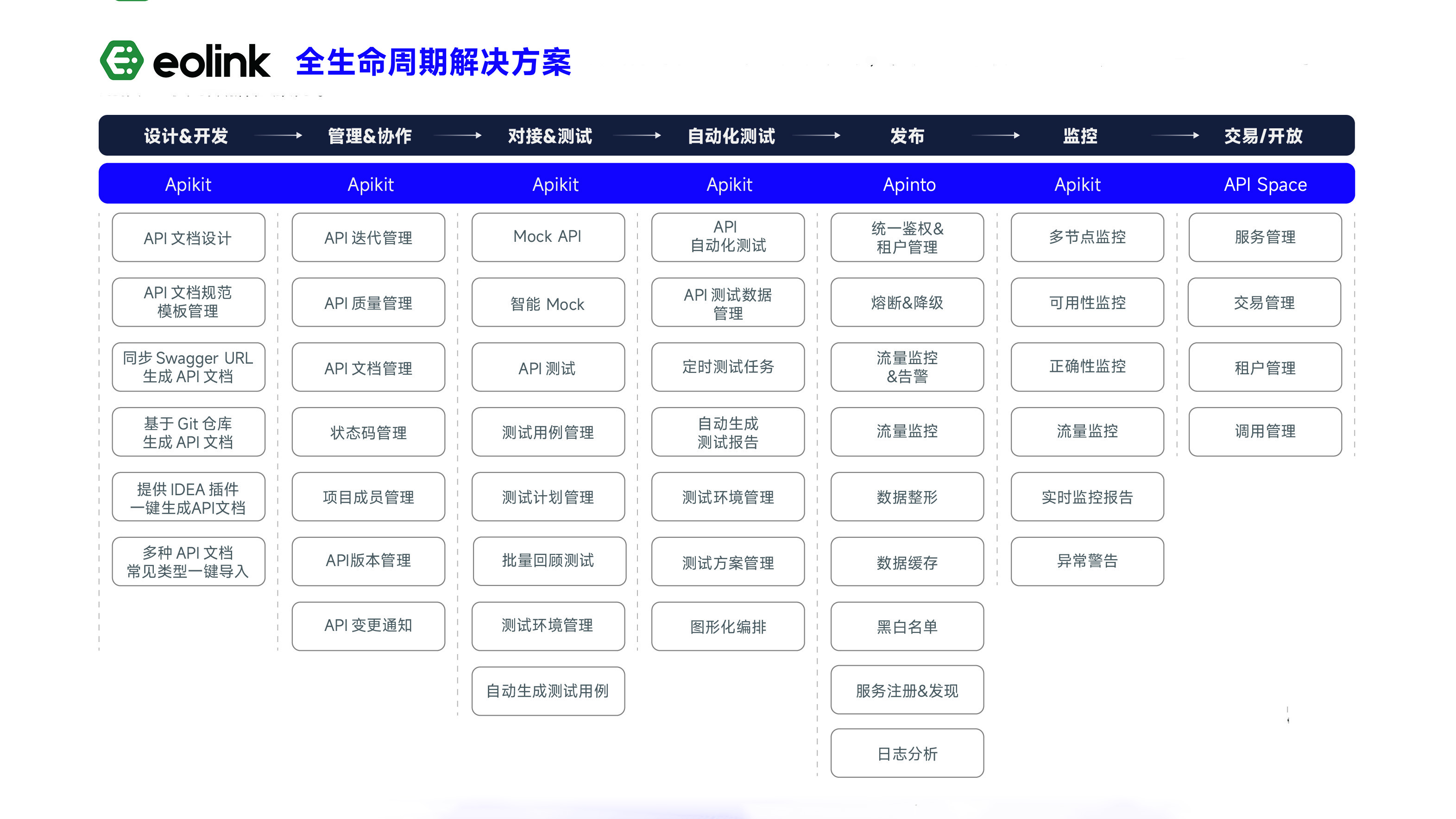
【下载】最佳实践手册结合Eolink在业内多年的实践经验,整理了API全生命周期中遇到的挑战及如何使用Eolink产品。
所有评论(0)